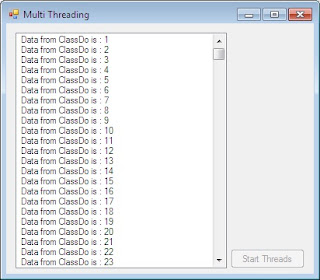
Threading
(This is one of the concept which I liked very much in .NET)
It gives the program to do many things simultaneously.Threading is used to divide many things at a same time but independently or dependently.
Here I will be telling in brief how threading is used in a small application using class and delegates.I will populate a listbox with numbers 0-500 bysing threads.
1) Create a windows application
2) Add a Form "FormMain"
3) Drop a button "ButtonStartThread"
3) Drop a button "ButtonStartThread"
4) Drop a Listbox "ListBoxNumbers"
5) Add a class "ClassDo"
6) In buttonClick write this
Private Sub ButtonStartThread_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ButtonStartThread.Click
Dim objClassDo As ClassDO
objClassDo = New ClassDO
AddHandler objClassDo.evtdisplayInLV, AddressOf evt_hndlr_displayInLV 'Event handler to handle the event raised from the classdo
objClassDo.startThread()
End Sub
Private Sub evt_hndlr_displayInLV(ByVal p_intVal As Integer)
'Call a delegate to handle this event.We do this to avoid cross thread operations
Me.Invoke(New del_displayLV(AddressOf hndlr_displayLV), p_intVal)
End Sub
'Declare a delegate
Private Delegate Sub del_displayLV(ByVal p_intVal As Integer)
Private Sub hndlr_displayLV(ByVal p_intVal As Integer)
'Populate the item in the listbox
ListBoxNumbers.Items.Add(p_intVal)
End Sub
8) In class Define these thingsImports System.Threading
Imports System.Windows
Public Class ClassDO
'Declare and event to raise in the main form
Public Event evtdisplayInLV(ByVal p_intVal As Integer)
'this function is called form the main form
Public Sub startThread()
'Call a thread here
Dim objTH As Thread
objTH = New Thread(AddressOf doStuff)
objTH.Start()
End Sub
'Procedure to be called by the thread
Private Sub doStuff()
Dim intMax As Integer = 500
Dim intVal As Integer = 0
While True
intVal += 1
If intVal > intMax Then
Exit While
End If
'Sleep the thread to view the progress
Thread.Sleep(10)
'Raise and event to the main form to display in the Listbox
RaiseEvent evtdisplayInLV(intVal)
End While
End Sub
End Class
No comments:
Post a Comment